Introduction
Pline (βPlugin interface languageβ) is a specification for describing command-line (CLI) programs and their interfaces, and its implementation as a lightweight web app. Pline generates interfaces from standardized descriptions, which allows users to create cross-platform GUIs without programming and to share or reuse existing interfaces. Pline has been designed for bioinformatics software, but a Pline interface can be written for any command-line executable.
A Pline command-line program description specifies a program and its input parameters in JSON format. You can download example interfaces as Pline plugins from the downloads page or write your own plugin JSON using the Pline JSON API.
Overview
A command-line program powered by a Pline interface consists of:
- the command-line program
- program description file
- Pline interface generator
- a server module
The file structure of a Pline package looks like this:
Pline_webapp
ββ pline.js //interface generator
ββ pline.css //interface styling rules
ββ index.html //example web page container
ββ pline_server.py //example server module
ββ plugins
ββ myProgram
ββ plugin.json //program description
ββ myProgram //executable binary
These files can be bundled together to form a standalone desktop application or used as part of a web page.
The Pline-specific interface generation files are plugin.json
, pline.js
and pline.css
files.
Rest of the package can be changed depending on the use case:
- the executable binary can be omitted when its global installation is available
- a custom web page can replace the
index.html
placeholder pline_server.py
is only executing ready-made commands and not tied to a specific Pline interface
How it works
The information flow in a Pline webapp:
- The interface generator
pline.js
reads the program descriptionplugin.json
and generates the interface into a web page - User fills the inputs and clicks the
RUN
button to launch the program - The server module
pline_server.py
runs the command received from the interface
Depending on the setup, the interface can control the executable on the local computer (standalone desktop app) or on a remote server (website integration). Correspondingly, the output files from the program runs can be accessed either directly in the file browser (local) or via download links (remote).
Installation
The downloads page includes a Pline program bundle for a number of command-line programs. The bundle can be used as a standalone web app or integrated to a website.
Standalone desktop app
- Installation:
- Download a Pline bundle from the plugins list
- Use:
- Launch the server module:
This will open a web browser window withpython pline_server.py #in the Pline directory
http://localhost:8000
to display the interface.
Standalone Pline website
You can use the included web page and server module to provide a Pline interface as a standalone web service.
- Installation:
- Download a Pline bundle to your server computer
- Launch the server module:
python pline_server.py #in the server
- Use:
- Point a web browser to your server web address
Integration to a website
Pline can draw interfaces to an existing web page and send the program commands to its backend server (instead of the Pline server module).
Installation:
- Include the Pline javascript file in your web page:
<script type='text/javascript' src='pline.js'></script>
Use:
- In the website: Give Pline a program description JSON and draw its interface:
//register a new program description let myPlugin = Pline.addPlugin('path/to/pluginFile.json'); //draw the interface into a HTML element myPlugin.draw('#container');
- In the server: The server receives a program command together with any iniput files:
//example payload from the interface { "program": "myProgram", "parameters": ["-flag", "-argName argValue", "-file inputFile.txt"], "files": { "inputFile.txt": "Some file content" } }
How to execute the command and deliver the result files, is up to the server. Example workflow is implemented in
pline_server.py
.
Multiple interfaces
Multiple program interfaces can be added to Pline:
- Download plugin package(s)
Plugin package includes interface JSON and the program binary
- Unzip to your Pline interfaces directory (by default Pline/plugins)
See the Pline file structure
- Register the plugins
//call for each added plugin
Pline.addPlugin("path/to/plugin/plugin.json")
This makes multiple program GUIs available in a single webapp. The interfaces can be drawn e.g. side-by-side or to separate web pages. In addition, all of the registered interfaces can be easily chained to a graphical pipeline:
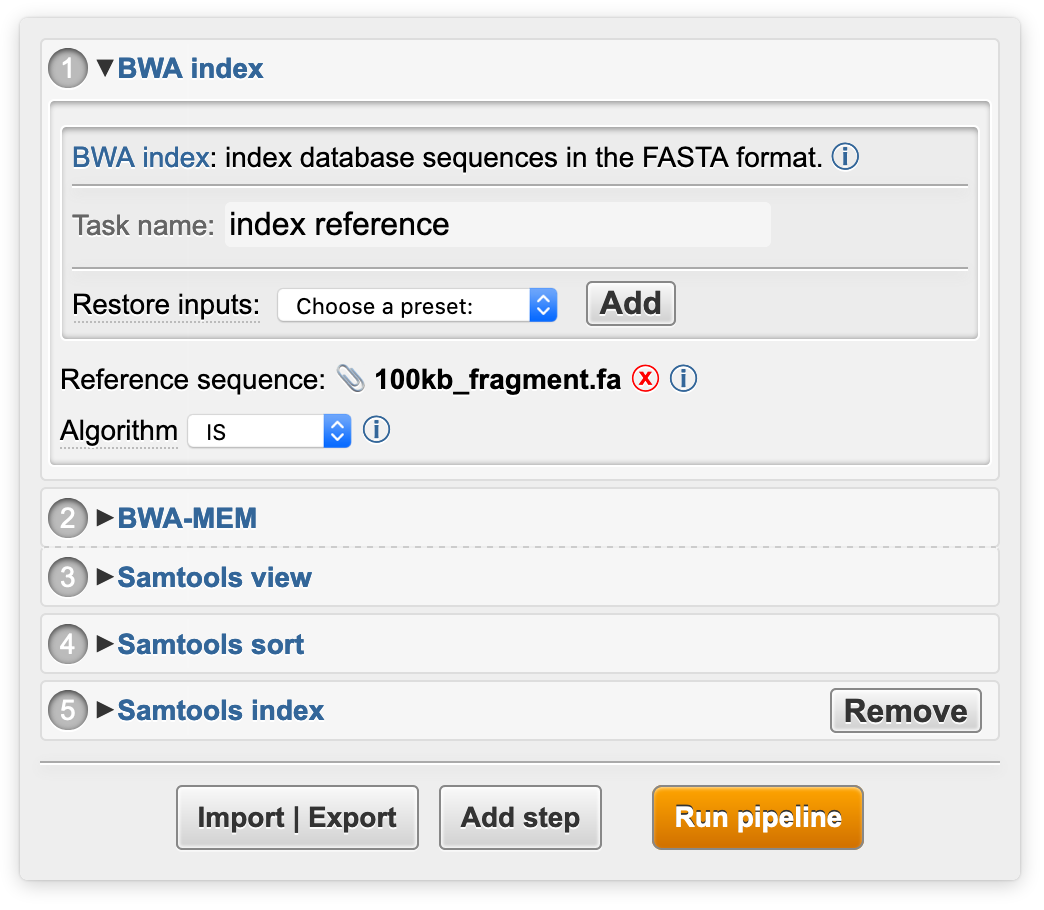
The buttons at the bottom allow to add program interfaces to the pipeline, export the pipeline to a JSON file and import stored pipelines. The example on the above image is available on the downloads page.
Advanced usage
Pline Javascript library exposes various settings and functions to modify the appearance and functionality of the generated program interfaces.
Interface settings
Pline.settings
object includes the adjustable global settings:
settings: {
sendmail: false, //enable email notifications (false|true|'pipelines'=only for pipelines)
email: '', //predefined email address
presets: true, //enable presets (stored plugin launch parameters)
UIcontainer: 'body', //default container element for plugin interfaces (CSS selector | DOM element)
pipelines: true, //enable pipelines (send multiple commands. false=show one plugin interface at a time)
pipes: true, //enable pipes in pipelines/commands (set false on unsopperted systems e.g. Windows)
sendAddress: '', //backend web server URL
sendData: {}, //default POST data, sent with each job (object: {key:value,...})
cleanup: false //true = remove interface after job has been sent to server
}
Extending Pline
Pline can be extended with custom functionality to intercept different lifecycle points in a plugin interface.
Extension functions can be added via Pline.extend(Name, Function)
with the following options:
Name | Intercepts | Use case | Function input |
---|---|---|---|
processPlugin | plugin import | Process plugin properties | Imported plugin JSON Object |
registerPlugin | plugin import | Add the plugin instance launcher to the web page | None. this refers to the plugin instance. |
processOption | parsing a program option | Implement custom JSON API | JSON Object for a single program option |
processPayload | constructing the program command | Send metadata to the server | Input: the payload Object being sent to the server |
Pline plugin API β